If you are using vuex in a small project, you can keep state, mutation, getters, and actions in a single file.
On the other hand, when developing a large project, we can divide vuex into modules based on categories, thereby keeping file sizes down, keeping the code cleaner, and increasing the chances of more than one developer being able to work on the project at the same time.
We can also separate state, mutation, getters, and actions within these modules. By using vuex modules, we want to increase manageability and understandability.
I will explain and show how to create modules per categories in this blog post. I will divide this blog post into two parts and explain how to exchange data between these modules in the second post, so as not to prolong it.
Modules
- auth: This module stores and processes data about user accounts.
- movies: This module stores and processes data about movies.
- notifications: This module stores and processes data about warnings, errors, etc.

Every module has its own state, mutations, getters, and actions files.
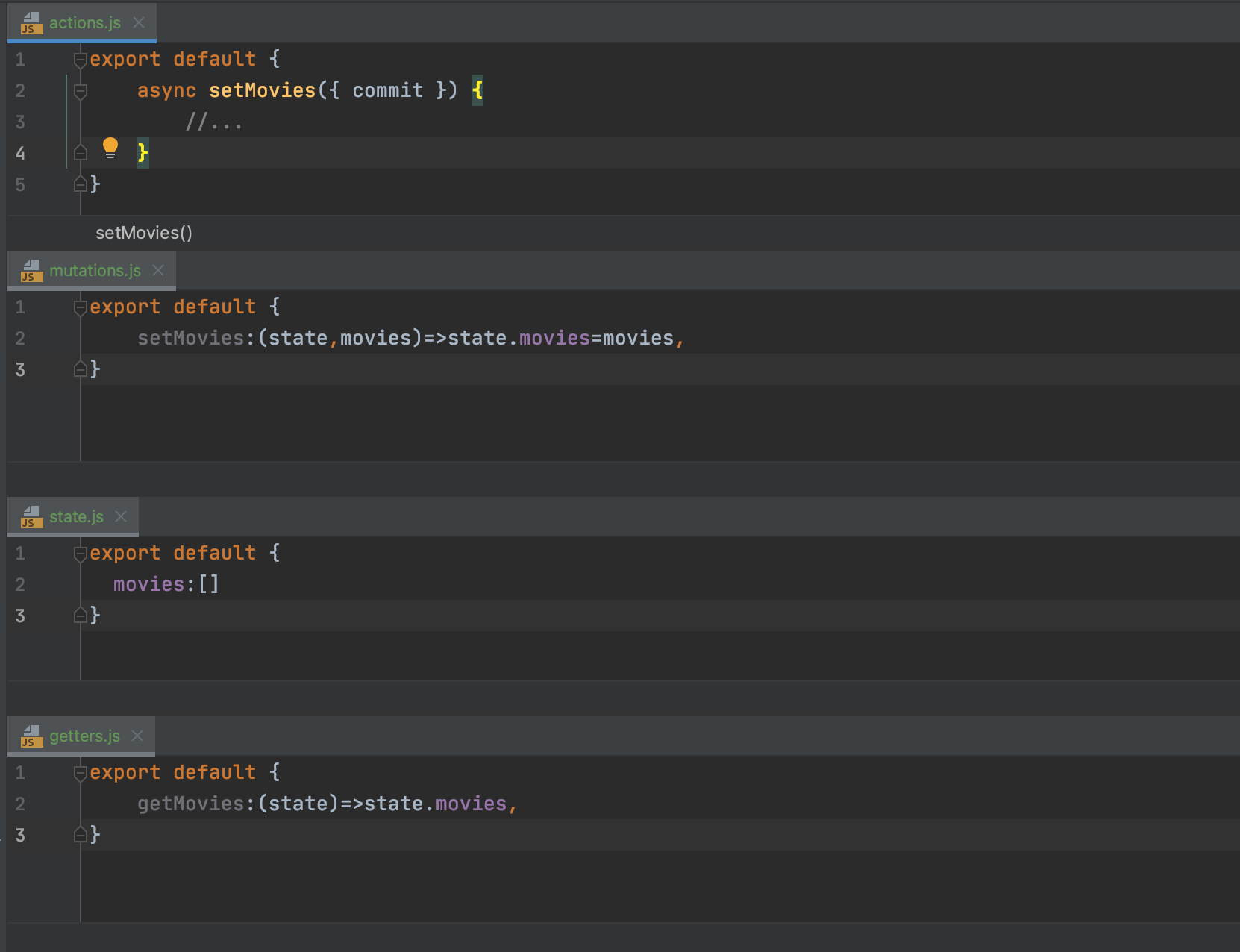
In each module, we create an index.js file that collects state, mutations, getters, and actions.
import state from './state';
import getters from './getters';
import mutations from './mutations';
import actions from './actions'
export default {
namespaced: true,
state,
getters,
mutations,
actions,
};
We import these modules and define them in the main store file index.js.
import { createStore } from 'vuex'
import auth from './modules/auth';
import movies from "./modules/movies";
import notifications from "./modules/notifications";
export default createStore({
modules: {
auth,
movies,
notifications
}
})
main.js uses it as follows:
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
import store from './store'
createApp(App).use(store).use(router).mount('#app')
As a result, vuex needs to be used more accurately to provide a more manageable and clean project.
The sample project is on my github account and the demo is on netlify.